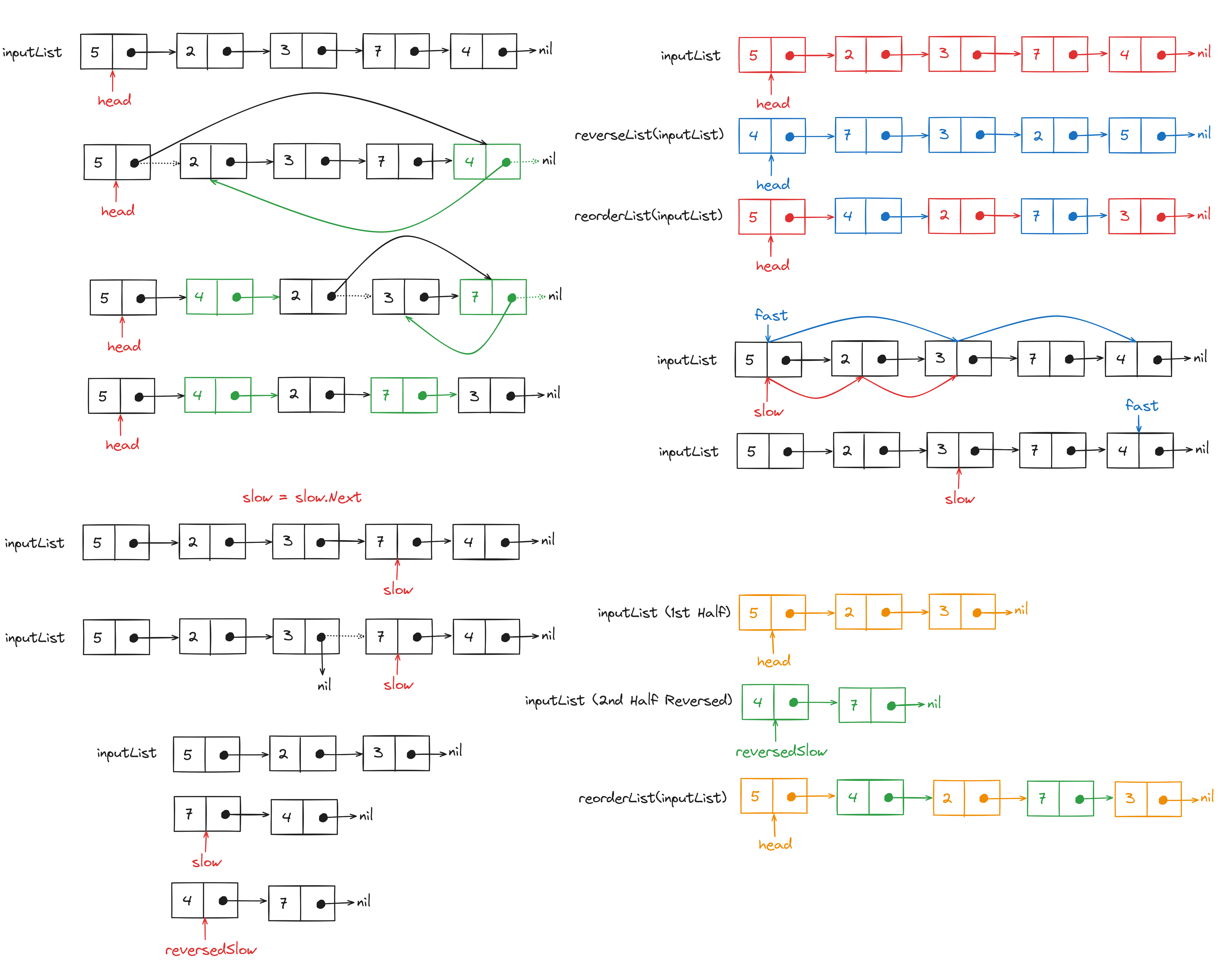
Reorder Linked Lists
Problem Statement We have to implement the reorderList function that takes the head node of a linked list as input and reorders its nodes in the format specified below. Given an input list like the following: $$Node_0 \rightarrow Node_1 \rightarrow Node_2 \rightarrow \dots \rightarrow Node_{(n-2)} \rightarrow Node_{(n-1)} \rightarrow Node_n$$ the reorderList function should reorder its nodes as: $$Node_0 \rightarrow Node_n \rightarrow Node_1 \rightarrow Node_{(n-1)} \rightarrow Node_2 \rightarrow Node_{(n-2)} \rightarrow \dots$$...