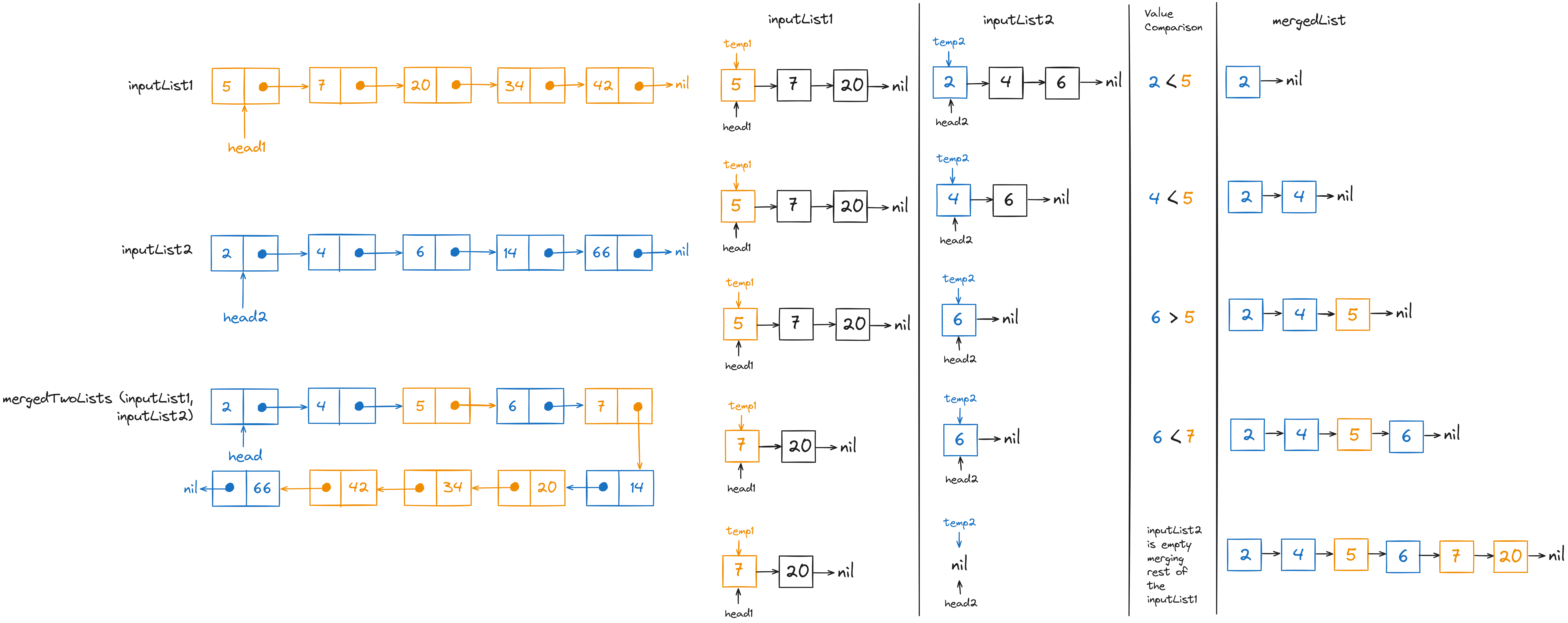
Merge Two Sorted Linked Lists
Problem Statement We have to implement the mergeTwoLists function that takes the head nodes of two sorted linked lists as input and returns the head node of the merged linked list as the output. Optimal Solution To merge both linked lists we can use the two-pointer approach by maintaining an iterator on both linked lists and comparing their values. The smaller value will be selected and inserted at the end of a new list....