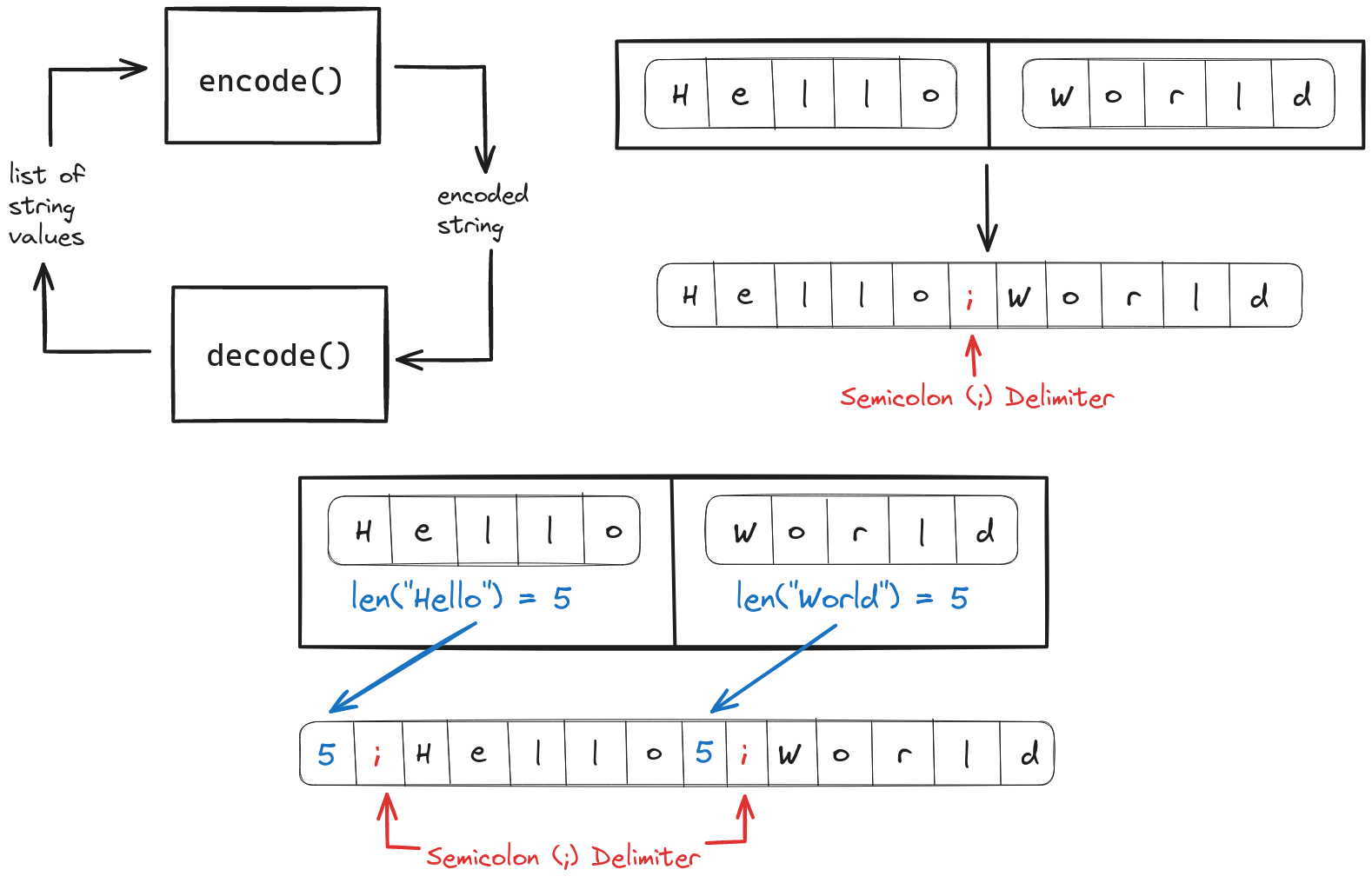
Encoding and Decoding Functions for Strings
Problem Statement We have to implement an encode function that takes a list of string values as input and returns a single encoded string that could be transmitted over a network. On the other side of the network, the decode function will take the encoded string as input and return the original list of string values as output. A delimiter could be used to differentiate between words. For example, ["Hello", "World"] could be encoded with comma delimiter (,) to "Hello,World"....